How to create Icon With text like home screen, here is my example screen:

To do something like this we need to use GridView and make The Icon
with text using the XML layout and then using LayoutInflater to read the
XML and put it into the Adapter. Lets begin with the icon.xml(we put it
into /res/layout/) that will be the view to show ImageView and Text
together.
03 |
android:id = "@+id/widget44" |
04 |
android:layout_width = "wrap_content" |
05 |
android:layout_height = "wrap_content" |
06 |
android:orientation = "vertical" |
07 |
android:layout_x = "201px" |
08 |
android:layout_y = "165px" |
09 |
android:gravity = "center_horizontal" > |
11 |
android:id = "@+id/icon_image" |
12 |
android:layout_width = "wrap_content" |
13 |
android:layout_height = "wrap_content" > |
16 |
android:id = "@+id/icon_text" |
17 |
android:layout_width = "wrap_content" |
18 |
android:layout_height = "wrap_content" |
19 |
android:text = "TextView" |
20 |
android:gravity = "center_horizontal" |
21 |
android:textColorHighlight = "#656565" > |
Here we put the ImageView and TextView
into Linear Layout. In line 7 and 8 we define how big is our icon with
layout_x and layout_y. Thats all, this icon.xml file next we will use in
the Adapter to fill in our GridView.
Lets go to our GridView XML. I name it main_switch.xml, this locate in /res/layout/.
01 |
<? xml version = "1.0" encoding = "utf-8" ?> |
04 |
android:id = "@+id/GridView01" |
05 |
android:layout_width = "fill_parent" |
06 |
android:layout_height = "fill_parent" |
07 |
android:padding = "10dp" |
08 |
android:verticalSpacing = "10dp" |
09 |
android:horizontalSpacing = "10dp" |
10 |
android:numColumns = "auto_fit" |
11 |
android:columnWidth = "60dp" |
12 |
android:stretchMode = "columnWidth" |
13 |
android:gravity = "center" > |
In this XML file we define the main layout of our screen, and how
will be the grid will be display. This will be load to our main
Activity.
Lets go to our Activity Class
01 |
public class MainSwitch extends Activity{ |
04 |
public void onCreate(Bundle savedInstanceState){ |
05 |
super .onCreate(savedInstanceState); |
06 |
setContentView(R.layout.main_switch); |
08 |
grid_main = (GridView)findViewById(R.id.GridView01); |
09 |
grid_main.setAdapter( new ImageAdapter( this )); |
11 |
public class ImageAdapter extends BaseAdapter{ |
13 |
public static final int ACTIVITY_CREATE = 10 ; |
14 |
public ImageAdapter(Context c){ |
18 |
public int getCount() { |
24 |
public View getView( int position, View convertView, ViewGroup parent) { |
27 |
if (convertView== null ){ |
28 |
LayoutInflater li = getLayoutInflater(); |
29 |
v = li.inflate(R.layout.icon, null ); |
30 |
TextView tv = (TextView)v.findViewById(R.id.icon_text); |
31 |
tv.setText( "Profile " +position); |
32 |
ImageView iv = (ImageView)v.findViewById(R.id.icon_image); |
33 |
iv.setImageResource(R.drawable.icon); |
Line 4 – line 10 : we overide onCreate() from the Activity class,
here we setContentView() to main_switch.xml, get the GridView and then
fill it with the ImageAdapter class that extend from BaseAdapter.
line 24-41 : we define the view that will display on the grid, we use
LayoutInflater to get view from icon.xml file that we already define
before. We also can manipulate both ImageView and TextView with what
parameter that we want to set, for example we can set the text or change
the ImageView Resource, etc.
line 18-21 : we set how many icon we want to display.
또는
<?xml version="1.0" encoding="utf-8"?>
<GridView
android:id="@+id/GridView01"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
xmlns:android="http://schemas.android.com/apk/res/android"
android:numColumns="auto_fit"
android:horizontalSpacing="8dp"
android:verticalSpacing="8dp"
android:columnWidth="130dp">
</GridView>
package com.MyImgGrid;
import android.app.Activity;
import android.content.Context;
import android.os.Bundle;
import android.view.*;
import android.widget.*;
public class MyImgGrid extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
GridView myGrid = (GridView) findViewById(R.id.GridView01);
myGrid.setAdapter(new ImageAdapter(this));
}
public class ImageAdapter extends BaseAdapter {
private Context mContext;
// 추가
public ImageAdapter(Context c) {
mContext = c;
}
// 수정
public int getCount() {
return mThumbs.length;
}
public Object getItem(int position) {
return null;
}
public long getItemId(int position) {
return 0;
}
// 수정
public View getView(int position, View convertView, ViewGroup parent) {
ImageView imgView;
if (convertView == null) {
imgView = new ImageView(mContext);
imgView.setLayoutParams(new GridView.LayoutParams(110, 110));
imgView.setScaleType(ImageView.ScaleType.CENTER_CROP);
imgView.setPadding(4, 4, 4, 4);
}
else {
imgView = (ImageView) convertView;
}
imgView.setImageResource(mThumbs[position]);
return imgView;
}
// 추가
private Integer[] mThumbs = {
R.drawable.f1, R.drawable.f2, R.drawable.f3, R.drawable.f4,
R.drawable.f5, R.drawable.f6
};
}
}
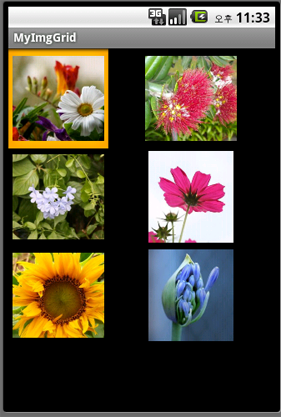