UIKeyboardTypeASCIICapable
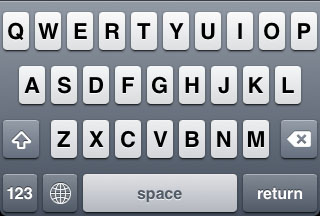
UIKeyboardTypeNumbersAndPunctuation
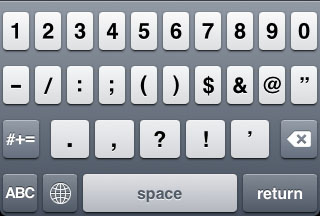
UIKeyboardTypeURL
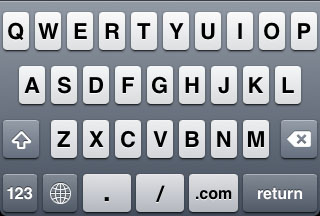
UIKeyboardTypeNumberPad
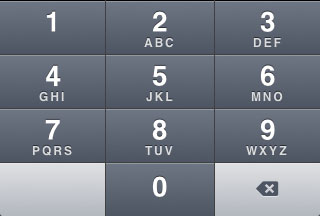
UIKeyboardTypePhonePad
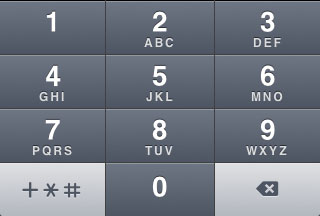
UIKeyboardTypeNamePhonePad
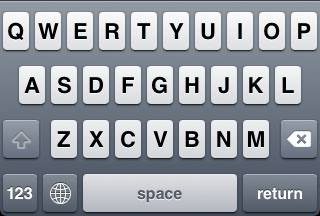
UIKeyboardTypeEmailAddress
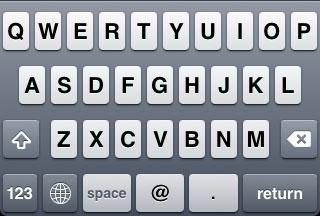
posted by : http://gprince.yolasite.com/index/uikeyboardtype
UIKeyboardTypeNumberPad and the missing "return" key | ![]() | ![]() | ![]() |
News - Development |
Written by Luzian Scherrer |
Tuesday, 21 April 2009 01:00 |
UPDATE 17 June 2010
If you have ever written an iPhone app that requires numeric input, then you surely know about the UIKeyboardTypeNumberPad. And if you have ever used that flavor of the iPhone's keyboard, then you surely know that it lacks one very important feature: The UIKeyboardTypeNumberPad does not have a "return" key. In fact every other keyboard type (except for the pretty similar UIKeyboardTypePhonePad) does offer the possibility to be dismissed by setting the returnKeyType property of the corresponding UITextInputTraits implementor. So how does one achieve the same effect with the number pad? We have found a workround! When looking at the number pad, you'll notice that there is an unused space on its bottom left. That's where we are going to plug in our custom "return" key. To make it short: take a screenshot, cut out the whole backspace key, flip it horizotally, clear its backspace symbol in Photoshop and overlay it with the text that we want on our “return” key. We’ve chosen to label it “DONE”. Now we have the image for our custom button’s UIControlStateNormal. Repeat the whole procedure (with a touched backspace key when taking the screenshot) to get a second image for our button’s UIControlStateHighlighted. Here’s the result:
Now back to coding. First we need to know when the number pad is going to be slided up on the screen so we can plug in our custom button before that happens. Luckily there’s a notification for exactly that purpose, and registering for it is as easy as: [[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillShow:) name:UIKeyboardWillShowNotification object:nil]; Don't forget to remove the observer from the notification center in the appropriate place once you're done with the whole thing: [[NSNotificationCenter defaultCenter] removeObserver:self]; Now we’re getting to the heart of it. All we have to do in the keyboardWillShow method is to locate the keyboard view and add our button to it. The keyboard view is part of a second UIWindow of our application as others have already figured out (see this thread). So we take a reference to that window (it will be the second window in most cases, so objectAtIndex:1 in the code below is fine), traverse its view hierarchy until we find the keyboard and add the button to its lower left: - (void)keyboardWillShow:(NSNotification *)note { // create custom button UIButton *doneButton = [UIButton buttonWithType:UIButtonTypeCustom]; doneButton.frame = CGRectMake(0, 163, 106, 53); doneButton.adjustsImageWhenHighlighted = NO; [doneButton setImage:[UIImage imageNamed:@"DoneUp.png"] forState:UIControlStateNormal]; [doneButton setImage:[UIImage imageNamed:@"DoneDown.png"] forState:UIControlStateHighlighted]; [doneButton addTarget:self action:@selector(doneButton:) forControlEvents:UIControlEventTouchUpInside]; // locate keyboard view UIWindow* tempWindow = [[[UIApplication sharedApplication] windows] objectAtIndex:1]; UIView* keyboard; for(int i=0; i<[tempWindow.subviews count]; i++) { keyboard = [tempWindow.subviews objectAtIndex:i]; // keyboard view found; add the custom button to it if([[keyboard description] hasPrefix:@"<UIKeyboard"] == YES) [keyboard addSubview:doneButton]; } } Voilà, that’s it! The empty space for our button starts at coordinate (0, 163) and has the dimensions (106, 53). The doneButton method has to be written now of course, but that’s not hard any more. Just make sure that you call resignFirstResponder on the text field that is being edited to have the keyboard slide down. We’re “DONE”. We have just discovered that the graphical design of the keyboard has slightly changed in the current iPhone OS 3.0 beta version. This means that the position and the image of our custom button will have to be adapted to that new style. The whole thing can be downloaded as Xcode project from us. |
'개발도구 > iOS - 아이폰 개발' 카테고리의 다른 글
[아이폰-펌] retain, release, autorelease, dealloc 에러 / ARC (Automatic Reference Counting) (0) | 2012.05.31 |
---|---|
[아이폰]_kCLLocatio error (0) | 2012.05.31 |
[펌-아이폰-비밀] TableView (0) | 2012.05.29 |
[아이폰] iOS 5 Storyboard and UITableViews Tutorial (0) | 2012.05.29 |
[아이폰] CLLocationDegrees < - > NSString (0) | 2012.05.25 |